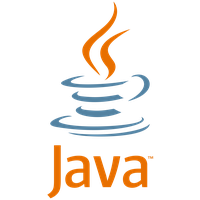

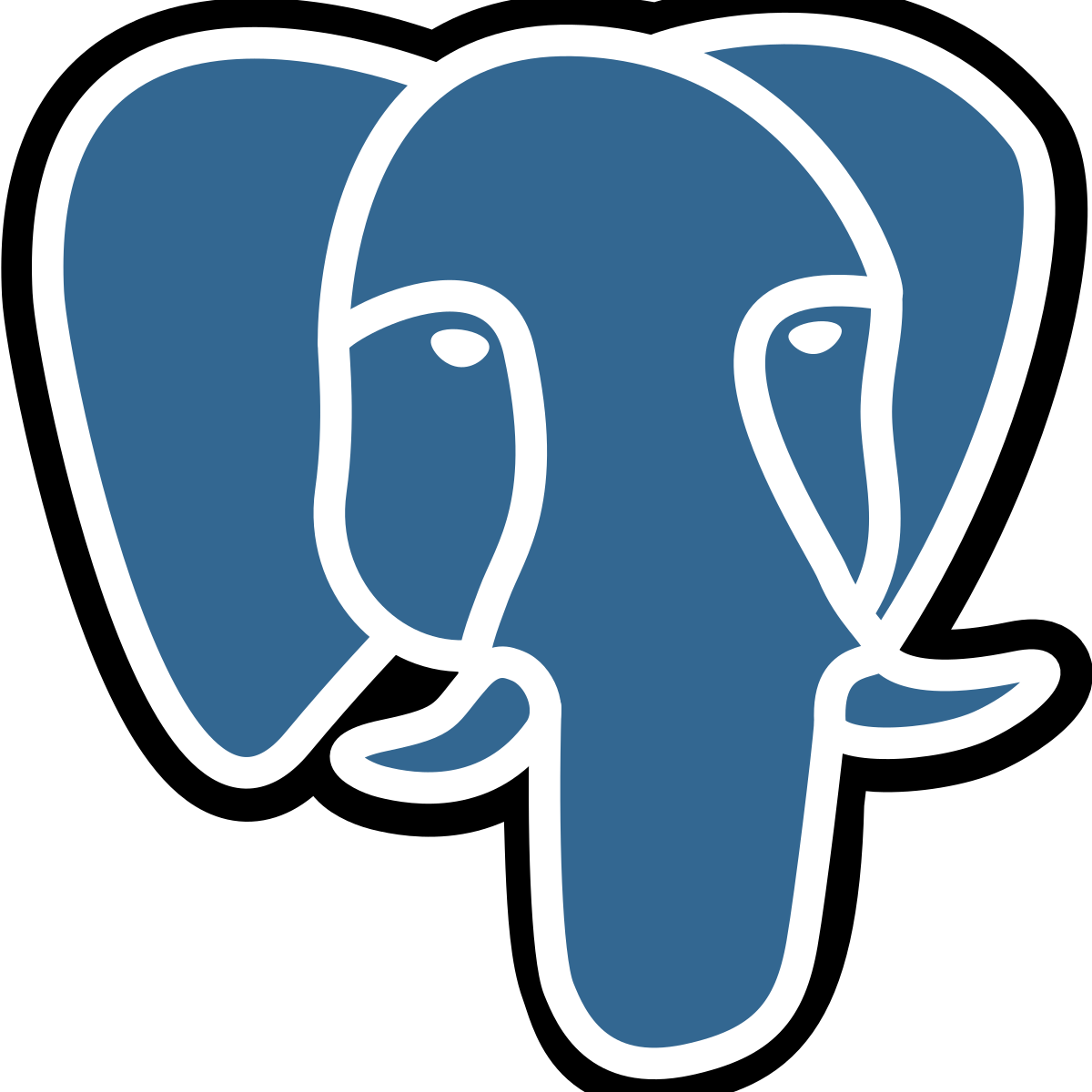
My team and I have been working on a lot of migrations off of Oracle and onto Google CloudSQL for Postgres / AlloyDB lately. One of the common things that I have been seeing in my performance tuning / migration activities is that Oracle handles certain application datatypes differently than PostgreSQL. For my most recent client, they used the “float” datatype extensively in their Java code and unfortunately, the PostgreSQL JDBC driver doesn’t convert that datatype like the Oracle JDBC driver does. The result of the datatype mismatch ends up as a full table scan in PostgreSQL whereas in Oracle it was using an index.
*** Note: This client did not use an ORM within their code. While I still need to test it, I am hopeful that this same issue will not manifest itself when using an ORM like SQLAlchemy (Python) or Hibernate (Java).
While in Oracle numbers are usually stored in the numeric datatype, you have many options within PostgreSQL to do the same thing:
- numeric (x)
- numeric (x,y)
- numeric
- smallint
- bigint
- int
Each serve a purpose and should be used with careful analysis. That said, don’t forget about the code! To demonstrate why, I modified a simple Java test harness I usually use to test connectivity / check SSL Encryption functionality during migrations to show what happens if the datatypes are not looked at carefully.
As you can see, in Oracle, the JDBC driver will convert any of the following types to “numeric“, the same query plan is also achieved using index range scans and no table scans. The output is below and Oracle Code is at the bottom of the post:
java -classpath /Users/shaneborden/Documents/java/ojdbc11.jar:/Users/shaneborden/Documents/java OracleJdbcTest Password: ******* ===== Database info ===== DatabaseProductName: Oracle DatabaseProductVersion: Oracle Database 19c Enterprise Edition Release 19.0.0.0.0 - Production Version 19.15.0.0.0 DatabaseMajorVersion: 19 DatabaseMinorVersion: 0 ===== Driver info ===== DriverName: Oracle JDBC driver DriverVersion: 21.8.0.0.0 DriverMajorVersion: 21 DriverMinorVersion: 8 ===== JDBC/DB attributes ===== Supports getGeneratedKeys(): true ===== Database info ===== ===== Query Plan - Cast Int to Numeric ===== Plan hash value: 423740054 ----------------------------------------------------------------------------------------------------------- | Id | Operation | Name | Rows | Bytes | Cost (%CPU)| Time | ----------------------------------------------------------------------------------------------------------- | 0 | SELECT STATEMENT | | 2 | 28 | 5 (0)| 00:00:01 | | 1 | TABLE ACCESS BY INDEX ROWID BATCHED| DATATYPE_TEST | 2 | 28 | 5 (0)| 00:00:01 | |* 2 | INDEX RANGE SCAN | DATATYPE_NUMBER_VAL | 2 | | 3 (0)| 00:00:01 | ----------------------------------------------------------------------------------------------------------- Predicate Information (identified by operation id): --------------------------------------------------- 2 - access("NUMBER_VAL"=:1) ===== Query Plan - Cast Long to Numeric ===== Plan hash value: 423740054 ----------------------------------------------------------------------------------------------------------- | Id | Operation | Name | Rows | Bytes | Cost (%CPU)| Time | ----------------------------------------------------------------------------------------------------------- | 0 | SELECT STATEMENT | | 2 | 28 | 5 (0)| 00:00:01 | | 1 | TABLE ACCESS BY INDEX ROWID BATCHED| DATATYPE_TEST | 2 | 28 | 5 (0)| 00:00:01 | |* 2 | INDEX RANGE SCAN | DATATYPE_NUMBER_VAL | 2 | | 3 (0)| 00:00:01 | ----------------------------------------------------------------------------------------------------------- Predicate Information (identified by operation id): --------------------------------------------------- 2 - access("NUMBER_VAL"=:1) ===== Query Plan - Cast Float to Numeric ===== Plan hash value: 423740054 ----------------------------------------------------------------------------------------------------------- | Id | Operation | Name | Rows | Bytes | Cost (%CPU)| Time | ----------------------------------------------------------------------------------------------------------- | 0 | SELECT STATEMENT | | 2 | 28 | 5 (0)| 00:00:01 | | 1 | TABLE ACCESS BY INDEX ROWID BATCHED| DATATYPE_TEST | 2 | 28 | 5 (0)| 00:00:01 | |* 2 | INDEX RANGE SCAN | DATATYPE_NUMBER_VAL | 2 | | 3 (0)| 00:00:01 | ----------------------------------------------------------------------------------------------------------- Predicate Information (identified by operation id): --------------------------------------------------- 2 - access("NUMBER_VAL"=:1) ===== Query Plan - Cast Double to Numeric ===== Plan hash value: 423740054 ----------------------------------------------------------------------------------------------------------- | Id | Operation | Name | Rows | Bytes | Cost (%CPU)| Time | ----------------------------------------------------------------------------------------------------------- | 0 | SELECT STATEMENT | | 2 | 28 | 5 (0)| 00:00:01 | | 1 | TABLE ACCESS BY INDEX ROWID BATCHED| DATATYPE_TEST | 2 | 28 | 5 (0)| 00:00:01 | |* 2 | INDEX RANGE SCAN | DATATYPE_NUMBER_VAL | 2 | | 3 (0)| 00:00:01 | ----------------------------------------------------------------------------------------------------------- Predicate Information (identified by operation id): --------------------------------------------------- 2 - access("NUMBER_VAL"=:1) ========================= Command successfully executed
However in PostgreSQL, the same statement where we convert “int” to “numeric” and “long” to “Numeric” an index scan is executed, however when casting “float” and “double” datatypes to numeric a table scan results. Similar behavior is seen when using the other PostgreSQL datatypes such as “smallint”, “bigint” and “int”. The output is shown below and the PostgreSQL Code is located at the bottom of the post:
java -classpath /Users/shaneborden/Documents/java/postgresql-42.5.0.jar:/Users/shaneborden/Documents/java PostgresJdbcTest Password: ******* ===== Database info ===== DatabaseProductName: PostgreSQL DatabaseProductVersion: 14.4 DatabaseMajorVersion: 14 DatabaseMinorVersion: 4 ===== Driver info ===== DriverName: PostgreSQL JDBC Driver DriverVersion: 42.5.0 DriverMajorVersion: 42 DriverMinorVersion: 5 ===== JDBC/DB attributes ===== Supports getGeneratedKeys(): true ===== Database info ===== Current Date from Postgres : 2022-12-27 16:25:41.493047-05 Client connected pid from Postgres : 17727 Postgres DB Unique Name from Postgres : mytpchdb Client connected hostname from Postgres : null Client connected application_name from Postgres : PostgreSQL JDBC Driver ===== Query Plan - Cast Int to Numeric ===== Index Scan using datatype_test_numeric_decimal on datatype_test (cost=0.43..6.47 rows=2 width=28) (actual time=0.057..0.060 rows=2 loops=1) Index Cond: (numeric_val = '10001'::numeric) Planning Time: 0.389 ms Execution Time: 0.085 ms ===== Query Plan - Cast Long to Numeric ===== Index Scan using datatype_test_numeric_decimal on datatype_test (cost=0.43..6.47 rows=2 width=28) (actual time=0.011..0.013 rows=2 loops=1) Index Cond: (numeric_val = '10001'::numeric) Planning Time: 0.126 ms Execution Time: 0.027 ms ===== Query Plan - Cast Float to Numeric ===== Seq Scan on datatype_test (cost=0.00..233334.01 rows=50000 width=28) (actual time=1050.733..2622.224 rows=2 loops=1) Filter: ((numeric_val)::double precision = '10001'::real) Rows Removed by Filter: 9999999 Planning Time: 0.094 ms Execution Time: 2622.273 ms ===== Query Plan - Cast Double to Numeric ===== Seq Scan on datatype_test (cost=0.00..233334.01 rows=50000 width=28) (actual time=1055.081..2629.634 rows=2 loops=1) Filter: ((numeric_val)::double precision = '10001'::double precision) Rows Removed by Filter: 9999999 Planning Time: 0.096 ms Execution Time: 2629.660 ms
As you can see, its very important to also ensure that your datatypes within your code are fully compliant with the destination RDBMS. The “double” and the “float” types within the Java code cause a table scan! While Oracle has become very forgiving with that over the years, PostgreSQL just isn’t there yet and you need to make sure that you adjust your code accordingly!
Code Samples:
Jar / Java Requirements:
- openjdk 11
- postgresql-42.5.0.jar
- ojdbc11.jar
Compile:
To compile the code:
Oracle: javac OracleJdbcTest.java
Postgres: javac PostgresJdbcTest.java
Oracle Java Test Harness:
Note: To execute the code follow the instructions in the comment block at the top of the code. The instructions to create the sample table objects are also contained within the same comment block.
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.util.Properties; import java.sql.*; /* * * Simple Java Program to connect Oracle database by using Oracle JDBC thin driver * Make sure you have Oracle JDBC thin driver in your classpath before running this program * @author javac OracleJdbcTest.java java -classpath /Users/shaneborden/Documents/java/ojdbc11.jar:/Users/shaneborden/Documents/java OracleJdbcTest Setup: CREATE TABLE tc.datatype_test ( number_decimal_val number(12,2), number_val number(12), random_val number(4)) TABLESPACE USERS; CREATE SEQUENCE tc.datatype_test_seq START WITH 1 INCREMENT BY 1 NOCACHE NOCYCLE; BEGIN FOR i in 1 .. 1000000 LOOP INSERT INTO tc.datatype_test VALUES ( tc.datatype_test_seq.nextval, floor(dbms_random.value(1, 1000000)), floor(dbms_random.value(1, 1000))); END LOOP; END; / CREATE INDEX tc.datatype_number_decimal_val on tc.datatype_test(number_decimal_val); CREATE INDEX tc.datatype_number_val on tc.datatype_test(number_val); */ public class OracleJdbcTest { public static void main(String args[]) throws SQLException, ClassNotFoundException { try { java.io.Console console = System.console(); Boolean dataTypeCheck = true; String sourceDatatType = "Numeric"; String inputPassword = new String(console.readPassword("Password: ")); Integer intQueryParam = 10001; Long longQueryParam = 10001L; Float floatQueryParam = 10001f; Double doubleQueryParam = 10001.0; /**Set URL of Oracle database server*/ String url = "jdbc:oracle:thin:@//192.168.1.105:1521/vboxncdb.localdomain.com"; String xPlanSql = "select * from table(dbms_xplan.display)"; /** properties for creating connection to Oracle database */ Properties props = new Properties(); props.setProperty("user", "datatypeTestUser"); props.setProperty("password", inputPassword); /** creating connection to Oracle database using JDBC*/ Connection conn = DriverManager.getConnection(url,props); DatabaseMetaData dbmd = conn.getMetaData(); System.out.println("===== Database info ====="); System.out.println(" DatabaseProductName: " + dbmd.getDatabaseProductName() ); System.out.println(" DatabaseProductVersion: " + dbmd.getDatabaseProductVersion() ); System.out.println(" DatabaseMajorVersion: " + dbmd.getDatabaseMajorVersion() ); System.out.println(" DatabaseMinorVersion: " + dbmd.getDatabaseMinorVersion() ); System.out.println("===== Driver info ====="); System.out.println(" DriverName: " + dbmd.getDriverName() ); System.out.println(" DriverVersion: " + dbmd.getDriverVersion() ); System.out.println(" DriverMajorVersion: " + dbmd.getDriverMajorVersion() ); System.out.println(" DriverMinorVersion: " + dbmd.getDriverMinorVersion() ); System.out.println("===== JDBC/DB attributes ====="); if (dbmd.supportsGetGeneratedKeys() ) System.out.println(" Supports getGeneratedKeys(): true"); else System.out.println(" Supports getGeneratedKeys(): false"); System.out.println("===== Database info ====="); String sql = "with session_data as ("; sql = sql + "select sysdate as current_day,SYS_CONTEXT ('USERENV', 'DB_UNIQUE_NAME') as db_name,SYS_CONTEXT ('USERENV', 'SERVICE_NAME') as service_name, "; sql = sql + "SYS_CONTEXT ('USERENV', 'HOST') as host, SYS_CONTEXT ('USERENV', 'IP_ADDRESS') as ip_address, SYS_CONTEXT('USERENV','SID') sid from dual) "; sql = sql + "select sd.current_day, sd.db_name, sd.service_name, sd.host, sd.ip_address, "; sql = sql + "sd.sid, nvl(sci.network_service_banner, 'Traffic Not Encrypted') network_service_banner "; sql = sql + "from session_data sd "; sql = sql + "left join v$session_connect_info sci on (sd.sid = sci.sid) "; sql = sql + "where sci.network_service_banner like '%Crypto-checksumming service adapter%'"; /** creating PreparedStatement object to execute query*/ PreparedStatement preStatement = conn.prepareStatement(sql); ResultSet result = preStatement.executeQuery(); while(result.next()) { System.out.println("Current Date from Oracle : " + result.getString("current_day")); System.out.println("Oracle DB Unique Name from Oracle : " + result.getString("db_name")); System.out.println("Oracle Connected Listener Service Name from Oracle : " + result.getString("service_name")); System.out.println("Client connected hostname from Oracle : " + result.getString("host")); System.out.println("Client connected ip_address from Oracle : " + result.getString("ip_address")); System.out.println("Client connected encryption info from Oracle : " + result.getString("network_service_banner")); } if (dataTypeCheck) if (sourceDatatType == "Numeric122") { sql = "EXPLAIN PLAN FOR "; sql = sql + "select * from tc.datatype_test where number_decimal_val = ?"; } else if (sourceDatatType == "Numeric") { sql = "EXPLAIN PLAN FOR "; sql = sql + "select * from tc.datatype_test where number_val = ?"; } System.out.println(""); System.out.println(""); System.out.println("===== Query Plan - Cast Int to "+ sourceDatatType +" ====="); /** creating PreparedStatement object to execute query*/ preStatement = conn.prepareStatement(sql); preStatement.setInt(1, intQueryParam); result = preStatement.executeQuery(); PreparedStatement xPlanStatement = conn.prepareStatement(xPlanSql); ResultSet xPlanResult = xPlanStatement.executeQuery(); while(xPlanResult.next()) { System.out.println(" " + xPlanResult.getString(1)); } System.out.println(""); System.out.println(""); System.out.println("===== Query Plan - Cast Long to "+ sourceDatatType +" ====="); preStatement.setLong(1, longQueryParam); result = preStatement.executeQuery(); xPlanStatement = conn.prepareStatement(xPlanSql); xPlanResult = xPlanStatement.executeQuery(); while(xPlanResult.next()) { System.out.println(" " + xPlanResult.getString(1)); } System.out.println(""); System.out.println(""); System.out.println("===== Query Plan - Cast Float to "+ sourceDatatType +" ====="); preStatement.setFloat(1, floatQueryParam); result = preStatement.executeQuery(); xPlanStatement = conn.prepareStatement(xPlanSql); xPlanResult = xPlanStatement.executeQuery(); while(xPlanResult.next()) { System.out.println(" " + xPlanResult.getString(1)); } System.out.println(""); System.out.println(""); System.out.println("===== Query Plan - Cast Double to "+ sourceDatatType +" ====="); preStatement.setDouble(1, doubleQueryParam); result = preStatement.executeQuery(); xPlanStatement = conn.prepareStatement(xPlanSql); xPlanResult = xPlanStatement.executeQuery(); while(xPlanResult.next()) { System.out.println(" " + xPlanResult.getString(1)); } System.out.println(""); System.out.println(""); conn.close(); System.out.println("========================="); System.out.println("Command successfully executed"); } catch(SQLException exp) { System.out.println("Exception: " + exp.getMessage()); System.out.println("SQL State: " + exp.getSQLState()); System.out.println("Vendor Error: " + exp.getErrorCode()); } } }
PostgreSQL Java Test Harness:
Note: To execute the code follow the instructions in the comment block at the top of the code. The instructions to create the sample table objects are also contained within the same comment block.
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.util.Properties; import java.sql.*; /* * * Simple Java Program to connect Postgres database by using Postgres JDBC thin driver * Make sure you have Postgres JDBC thin driver in your classpath before running this program * @author java -classpath /Users/shaneborden/Documents/java/postgresql-42.5.0.jar:/Users/shaneborden/Documents/java PostgresJdbcTest Setup: CREATE TABLE datatype_test ( int_val int, bigint_val bigint, numeric_val numeric(12), numeric_decimal_val numeric(12,2), smallint_val smallint); INSERT INTO datatype_test VALUES ( generate_series(0,10000000), generate_series(0,10000000), floor(random()*10000000), random()*10000000, floor(random()* (32765-1 + 1) + 1) ); SET SESSION max_parallel_maintenance_workers TO 4; SET SESSION maintenance_work_mem TO '2 GB'; CREATE INDEX datatype_test_int on datatype_test(int_val); CREATE INDEX datatype_test_bigint on datatype_test(bigint_val); CREATE INDEX datatype_test_numeric on datatype_test(numeric_val); CREATE INDEX datatype_test_numeric_decimal on datatype_test(numeric_val); CREATE INDEX datatype_test_smallint on datatype_test(smallint_val); */ public class PostgresJdbcTest { public static void main(String args[]) throws SQLException, ClassNotFoundException { try { java.io.Console console = System.console(); Boolean dataTypeCheck = true; String sourceDatatType = "Numeric"; String inputPassword = new String(console.readPassword("Password: ")); Integer intQueryParam = 10001; Long longQueryParam = 10001L; Float floatQueryParam = 10001f; Double doubleQueryParam = 10001.0; /**Set URL of Postgres database server*/ String url = "jdbc:postgresql://localhost:6000/mytpchdb"; /** properties for creating connection to Postgres database */ Properties props = new Properties(); props.setProperty("user", "postgres"); props.setProperty("password", inputPassword); /** creating connection to Postgres database using JDBC*/ Connection conn = DriverManager.getConnection(url,props); DatabaseMetaData dbmd = conn.getMetaData(); System.out.println("===== Database info ====="); System.out.println(" DatabaseProductName: " + dbmd.getDatabaseProductName() ); System.out.println(" DatabaseProductVersion: " + dbmd.getDatabaseProductVersion() ); System.out.println(" DatabaseMajorVersion: " + dbmd.getDatabaseMajorVersion() ); System.out.println(" DatabaseMinorVersion: " + dbmd.getDatabaseMinorVersion() ); System.out.println("===== Driver info ====="); System.out.println(" DriverName: " + dbmd.getDriverName() ); System.out.println(" DriverVersion: " + dbmd.getDriverVersion() ); System.out.println(" DriverMajorVersion: " + dbmd.getDriverMajorVersion() ); System.out.println(" DriverMinorVersion: " + dbmd.getDriverMinorVersion() ); System.out.println("===== JDBC/DB attributes ====="); if (dbmd.supportsGetGeneratedKeys() ) System.out.println(" Supports getGeneratedKeys(): true"); else System.out.println(" Supports getGeneratedKeys(): false"); System.out.println("===== Database info ====="); String sql = "select now() as current_day,current_database() as db_name, "; sql = sql + "client_hostname as host, application_name, pid from pg_stat_activity "; sql = sql + " where pid = pg_backend_pid() "; /** creating PreparedStatement object to execute query*/ PreparedStatement preStatement = conn.prepareStatement(sql); ResultSet result = preStatement.executeQuery(); while(result.next()) { System.out.println(" Current Date from Postgres : " + result.getString("current_day")); System.out.println(" Client connected pid from Postgres : " + result.getString("pid")); System.out.println(" Postgres DB Unique Name from Postgres : " + result.getString("db_name")); System.out.println(" Client connected hostname from Postgres : " + result.getString("host")); System.out.println(" Client connected application_name from Postgres : " + result.getString("application_name")); } if (dataTypeCheck) if (sourceDatatType == "Int") { sql = "EXPLAIN (ANALYZE, COSTS)"; sql = sql + "select * from datatype_test where int_val = ?"; } else if (sourceDatatType == "Bigint") { sql = "EXPLAIN (ANALYZE, COSTS)"; sql = sql + "select * from datatype_test where bigint_val = ?"; } else if (sourceDatatType == "Numeric") { sql = "EXPLAIN (ANALYZE, COSTS)"; sql = sql + "select * from datatype_test where numeric_val = ?"; } else if (sourceDatatType == "Numeric122") { sql = "EXPLAIN (ANALYZE, COSTS)"; sql = sql + "select * from datatype_test where numeric_val = ?"; } else if (sourceDatatType == "Smallint") { sql = "EXPLAIN (ANALYZE, COSTS)"; sql = sql + "select * from datatype_test where smallint_val = ?"; } Statement stmt = conn.createStatement(); stmt.execute("SET max_parallel_workers_per_gather = 0"); System.out.println(""); System.out.println(""); System.out.println("===== Query Plan - Cast Int to "+ sourceDatatType +" ====="); /** creating PreparedStatement object to execute query*/ preStatement = conn.prepareStatement(sql); preStatement.setInt(1, intQueryParam); result = preStatement.executeQuery(); while(result.next()) { System.out.println(" " + result.getString(1)); } System.out.println(""); System.out.println(""); System.out.println("===== Query Plan - Cast Long to "+ sourceDatatType +" ====="); preStatement.setLong(1, longQueryParam); result = preStatement.executeQuery(); while(result.next()) { System.out.println(" " + result.getString(1)); } System.out.println(""); System.out.println(""); System.out.println("===== Query Plan - Cast Float to "+ sourceDatatType +" ====="); preStatement.setFloat(1, floatQueryParam); result = preStatement.executeQuery(); while(result.next()) { System.out.println(" " + result.getString(1)); } System.out.println(""); System.out.println(""); System.out.println("===== Query Plan - Cast Double to "+ sourceDatatType +" ====="); preStatement.setDouble(1, doubleQueryParam); result = preStatement.executeQuery(); while(result.next()) { System.out.println(" " + result.getString(1)); } System.out.println(""); System.out.println(""); conn.close(); System.out.println("========================="); System.out.println("Command successfully executed"); } catch(SQLException exp) { System.out.println("Exception: " + exp.getMessage()); System.out.println("SQL State: " + exp.getSQLState()); System.out.println("Vendor Error: " + exp.getErrorCode()); } } }